Table of Contents
Introduction
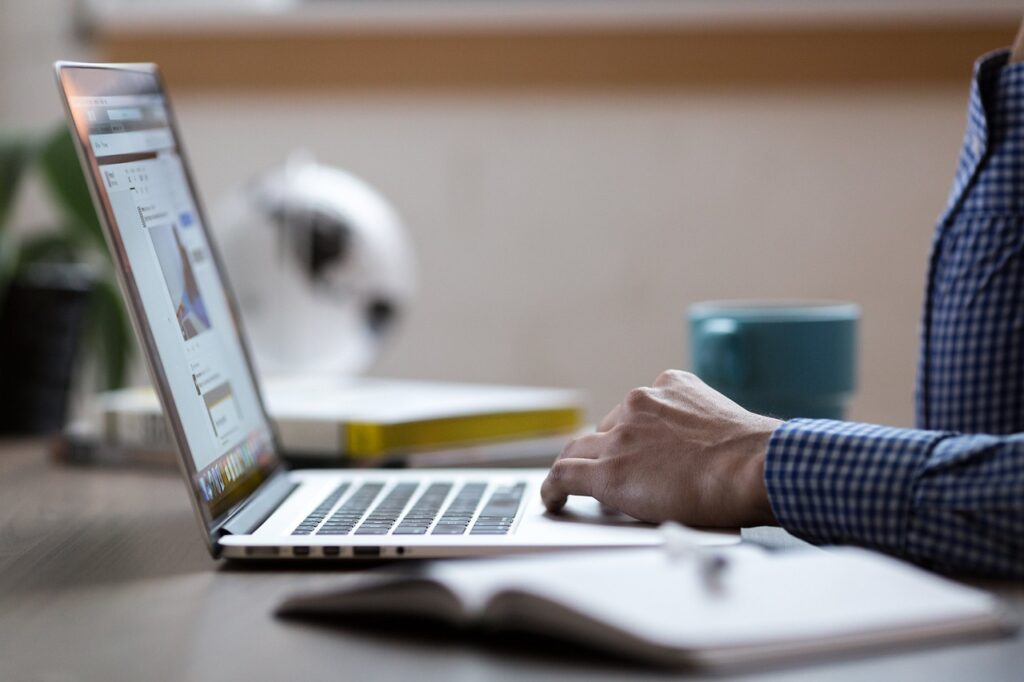
Hello Everybody, Today we are going to learn about methods in java. This article is going to be very fun and full of knowledge. I a going to teach you everything about methods for example why we need methods in java ? Its types ? User defined methods, Syntax to define a method and many more. So just read this full article and I’ll promise you never going to forgot about methods. So let’s get started.
Methods : Definition
So a method can be defined as a set of instructions which can be defined once and can be use multiple times just by calling its name. A method can take one or more parameters and can also return a value as a result. A methods can be public, private and protected as well in java. Methods are also know as functions in java.
Why we need methods in java ?
So we are learning about methods but have you ever thought of this that why we need methods in java ? So let’s find out answer of this question with an example let’s suppose I want to perform addition of two variables in java and I want to calculate the addition multiple times in my entire programme. So how can we achieve this ? Do you remember what the definition of method says ? it says “A method can be defined as a set of instructions which can be defined once and can be use multiple times just by calling its name” So our problem statement is also the same we want to perform addition but multiple times in our programme. So to counter this problem we can use methods.
Syntax of an method
Basic syntax of methods in java
/*
This is the syntax of a method
modifier return-type (parameters)
{
// This is body
}
*/
// This is real representation of method
private void Addition(){
}
Let’s understand each and everything about syntax used in method declaration.
- Modifier : modifier tells the compiler is this function is going to be private, public or protected.
- Return-type : return-type tell the compiler is the method return something as a result or not. return-type can be a list, array-list, int, string etc. If we do not want to return anything as a result then we can define the return-type as void.
- Parameters : Parameters are the value which a function takes to perform operation on it. For example if I want to add two variable by using a function. Then first I am going to define to int type parameters and on calling the function I am going to send two integer type values to the function. So that I can get Addition of the two variables.
- Curly brackets {} : These curly brackets are the body of the function. In these curly bracket we can write our code which we want our function to perform when it gets called.
Different representation of methods
Methods with no parameter and return value
public class Main {
// main is also an pre-defined method
public static void main(String args[]){
// Object creation of Main class of that we can call
Main obj = new Main();
obj.PrintGoodMorning();
}
private void PrintGoodMorning(){
System.out.println("Good Morning");
}
}
Methods with return type but no parameter
public class Main {
// main is also an pre-defined method
public static void main(String args[]){
// Object creation of Main class of that we can call
Main obj = new Main();
// return "Good Morning" from PrintGoodMorning method is getting stored in message variable
String message = obj.PrintGoodMorning();
System.out.println(message);
}
private String PrintGoodMorning(){
return "Good Morning";
}
}
Method with parameter but no return type
public class Main {
public static void main(String args[]){
Main obj = new Main();
// Sending Good Morning String as an parameter So we can use it later on.
String message = obj.PrintGoodMorning("Good Morning");
}
private void PrintGoodMorning(String message){
System.out.println(message);
}
}
Method with parameter and return type
public class Main {
public static void main(String args[]){
Main obj = new Main();
// Sending Good Morning String as an parameter So we can use it later on.
String message = obj.PrintGoodMorning("Good Morning");
System.out.println(message);
}
private String PrintGoodMorning(String message){
return message;
}
}
So here is the interesting part all these different ways just print one message and that is “Good Morning” shocked right ? Actually these are the representation about how you can write methods in different ways according to your need. In some cases basic function is enough but sometimes the value passed to method is dynamic then we will use parameters and if we want our function to return some result after calculation then we will define a return-type. So it’s all depend upon the requirement of the programmer.
Types of methods
- User-defined method
- Pre-defined method
Pre-defined method
As the name suggests pre-defined methods are those which are already provided by pre-defined classes. You can directly use those according to your need. There are a lot of pre-defined methods provided by java libraries some of the examples are mentioned below in the code
public class Main {
// main is also an pre-defined method
public static void main(String args[]){
// printl is predefined funtion
System.out.println("Hey this is predefined method");
// sqrt is also an predefined funtion
System.out.println(Math.sqrt(35) +" This result is also given by predefined method called sqrt");
}
}
User-defined method
As the name suggests user-defined functions are those who are defined by the programmer. Programmer have all the power from defining name, return-type, functionality etc. The basic example to understand user defined function by code is Example 1.0
public class Main {
// main is also an pre-defined method
public static void main(String args[]){
// Object creation of Main class of that we can call PrintGoodMorning method
Main obj = new Main();
obj.PrintGoodMorning();
}
private void PrintGoodMorning(){
System.out.println("Good Morning");
}
}
So in above code we created a function called PrintGoodMorning. By calling this function we can print Good Morning. To print Good Morning we need to call this function and we can call this function by creating an object of Class Main because PrintGoodMorning method is defined in Main class. With the help of Main class object we can now call PrintGoodMorning function.
Methods in Java can also be classified into the following types:
- Static method
- Instance method
- Abstract method
Static method
Static methods are those who directly belongs to the class not to the instance of the class. We can directly call a static method without making an instance of the class. The only condition we have to define a static method is create a method this static keyword. The example code of static method is Example 1.1
public class Main {
// main is also an pre-defined method
public static void main(String args[]){
/*
Now see we don't need to make an object of Main class. We directly called PrintGoodMoring method
Because it's static method.
*/
PrintGoodMorning();
}
private static void PrintGoodMorning(){
System.out.println("Good Morning");
}
}
Instance method
So in above example 1.0 of method you might have think why we are creating object of the class to call a function ? Why we cannot directly call it by it name. So the simple answer is that because the method PrintGoodMorning we defined is non static method and that falls under the category of instance based method. In Instance based method the method directly does not belongs to the class and can be called by creating and calling the object of the class in which the method is declared.
public class Main {
// main is also an pre-defined method
public static void main(String args[]){
// Object creation of Main class of that we can call PrintGoodMorning method
Main obj = new Main();
obj.PrintGoodMorning();
}
private void PrintGoodMorning(){
System.out.println("Good Morning");
}
}
Abstract method
Abstract methods are those who does not require it’s body to be declared by the time we are defining it. We can define the body of an abstract method later on. Abstract method can be declared by using abstract keyword and there is one more hard rule that abstract method can only be declared with in the abstract class. I know it sounds quite confusing but let’s just understand it with example code
abstract class AbstractClass{ // Abstarct class
abstract void show(); // Abstract method
}
public class Main extends AbstractClass{
void show(){
System.out.println("This is show method");
}
public static void main(String[] args) {
AbstractClass obj = new Main();
obj.show();
}
}
So as we can see in above example we declare our method with abstract keyword in abstract class and we provided no body to the method. But if you note later on we provided body to our show method in Main class and then with the help of object we called the method in main function to perform print “This is show method”. If you observe we extended our Main class with AbstractClass using extend keyword. Why we did this ? This is a whole new topic but In short we extended our Main class with Abstract class so that Main class can also use the properties of AbstractClass.
Conclusion
I hope you guys understood the basic and advance of a methods in java. I tried my best to explain you guys each and everything about methods. Still if you guys have any doubts then comment section is all your ask anything related to you doubts. If you have any suggestions you can contact us at suggestions@thecseguide.com.