Table of Contents
Introduction
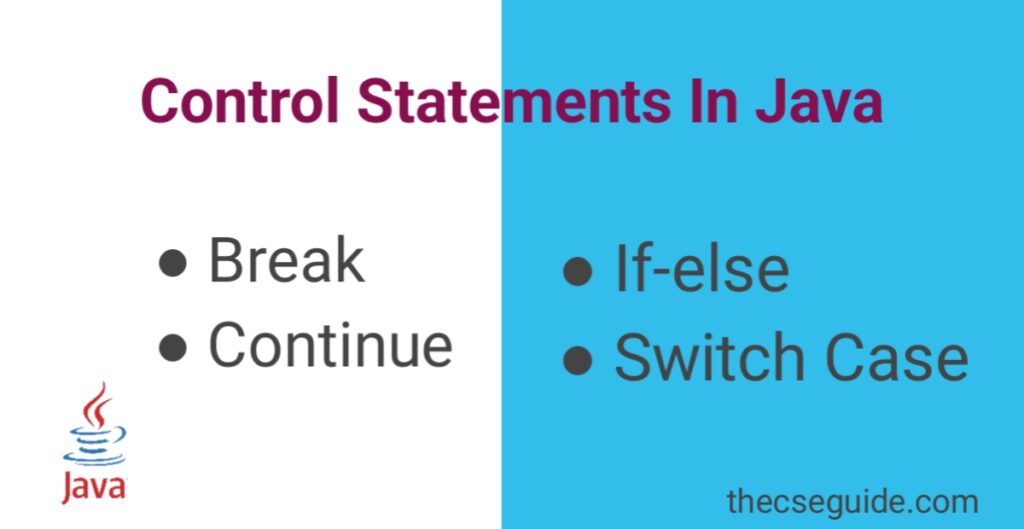
Hello Everyone, Today we are going to learn what are flow control statements in java ? As the name suggests these are used to control the flow of the code. I will also teach you the syntax and usage of the flow control state. If you learned the usage of flow control statements then you can make a code which can be effective in term of taking decisions. So let’s get started and learn this interesting topic with ease.
Types of Flow control statements
- If statements
- Switch Case Statements
- Jump Statements
- Loops Statements
These 3 are the main types of flow control statements. You have to master only these three to learn the concept of flow control statements. Let first start with If statements.
If Statement
If statement is quite straightforward and easy to implement. Code written in If statement is only runs when a condition is fulfilled. To understand a If statement let’s understand its syntax first.
Syntax
if (condition){
// This code will run when the condition is true
}
If statement code
public class Main
{
public static void main(String[] args) {
// This is a boolean which will decide is if block going to run or not
boolean iWantToStudyScience = true;
if (iWantToStudyScience){
// This code will run when the condition is true
System.out.println("Study Science");
}
}
}
If-else Statement
So to understand the if-else statement let’s compare this with real life scenario. For example you thought today I am going to study science for 2 hours but for some reason you didn’t get the chance to study science. So then you decide if I am not able to study science today then I will study maths and if else works on the same principle. By using if-else statement we make sure if the code inside if block doesn’t matches the condition then we have else code which always gonna run when our condition is false. Let’s understand with entire thing with practical code.
Syntax
if (condition){
// This code will run when the condition is true
}
else{
// This code will run when the condition is false
}
If-else statement code
public class Main
{
public static void main(String[] args) {
// This is a boolean which will decide is if block going to run or else block
boolean iWantToStudyScience = false;
if (iWantToStudyScience){
// This code will run when the condition is true
System.out.println("Study Science");
}else{
// This code will run when the condition is false
System.out.println("Study Maths");
}
}
}
If-else-if ladder statement
So the third type of if-else statement is the ladder statement. As the name suggest it work the same like a ladder. It checks multiple condition statements one by one and if any condition is true then it will run the code block of that condition which is true. So let’s first know what’s the need of if-else-if ladder statement ? If you observe in above if-else statement we only have one chance to verify if the condition is true or not.
if the condition is not true then we jump on the else block to run the specific instrictions. But what if I say I have more than 1 condition to check? Now in this case we can use if-else-if ladder statement. After all this explanation let’s see the syntax and code for if-else-if statement.
Syntax
if (condition){
}
else if (condition){
}
else if(condition){
}
else{
}
If-else-if statement code
public class Main
{
public static void main(String[] args) {
int age = 18;
if (age == 20){
System.out.println("Age is 20");
}
else if (age == 18){
System.out.println("Age is 18");
}
else if(age = 21){
System.out.println("Age is 21");
}
else{
System.out.println("Your age is not 20,18 and 21");
}
}
}
In above code we took an int variable called age and assign it a value of 18. Now it is possible that the user age can be 20, 21, 18 or something else. So we are checking if user age is 18, 20 and 21 then we will display a specific message according to their age. If the user age is not 18,20 and 21 then we will display user a message that “Your age is not 20,18 and 21”.
Nested If-else statement
Let’s understand what is Nested if-else statement. What If I say to you that check a condition after a certain condition is true. In that case what you gonna do ? Obviously we cannot use if-else-if ladder statement, if statement or even a if-else statement because we have to check a condition only after when a certain condition is true. So in this case we will use nested If-else statement. So let’s first take a look to syntax then we will look into the code.
Syntax
if (condition){
// Nested if condition
if(condition){
}
}
else{
// Nested if condition under else block
if(condition){
}
}
Nested if-else code
public class Main
{
public static void main(String[] args) {
boolean IsDoorLocked = true;
if (IsDoorLocked){
System.out.println("Door is locked let's open it.");
// Nested if condition
IsDoorLocked = false;
if(IsDoorLocked){
System.out.println("Door is still locked");
}else{
System.out.println("Door is unlocked");
}
}
}
}
So what I did above first I defined a boolean called “IsDoorLocked” and the initial value of this variable is true and then we used a if statement to check if the IsDoorLocked and when we enter inside the if statement block because our IsDoorLocked is true then we will assign IsDoorLocked variable a value of false. Then again inside that if statement we are checking by using a if statement if the IsDoorLocked true but this time it will execute the else part because we assigned the false value to IsDoorLocked variable.
Switch Case Statement
After learning if-else statement. Now it’s time to learn Switch case statement. In switch case we use switch keyword to tell the compiler that we want our case to be execute on the bases of expression provided by the switch keyword. We use case keyword to define the particular case for the given expression and break keyword is used to tell the compiler that we are done and now exit from this case and run further code. Also in below code you will observer a keyword called default. So default keyword works in the same manner as the else do. For example if on given expression none of the case matches then default part will be run.
Syntax
switch (expression) {
case value1:
// Code to execute if expression equals value1
break;
case value2:
// Code to execute if expression equals value2
break;
// You can have any number of case statements
default:
// Code to execute if no cases match
}
Switch case code
int day = 3;
String dayName;
switch (day) {
case 1:
dayName = "Sunday";
break;
case 2:
dayName = "Monday";
break;
case 3:
dayName = "Tuesday";
break;
case 4:
dayName = "Wednesday";
break;
case 5:
dayName = "Thursday";
break;
case 6:
dayName = "Friday";
break;
case 7:
dayName = "Saturday";
break;
default:
dayName = "Invalid day";
break;
}
System.out.println(dayName);
Break keyword
The main purpose of using the break
keyword is to tell the compiler that we are done with the ongoing execution within the current loop or switch statement. The break
keyword stops the current execution and allows the program to proceed with the subsequent code outside the loop or switch statement.
Syntax : Switch Statement
switch (expression) {
case value1:
// Code to execute if expression equals value1
break;
case value2:
// Code to execute if expression equals value2
break;
// You can have any number of case statements
default:
// Code to execute if none of the cases match
break;
}
Syntax : For loop
for (initialization; condition; update) {
// Code to execute in each iteration
if (someCondition) {
break; // Exit the loop if someCondition is true
}
}
Syntax : While loop
while (condition) {
// Code to execute in each iteration
if (someCondition) {
break; // Exit the loop if someCondition is true
}
}
Syntax : Do While loop
do {
// Code to execute in each iteration
if (someCondition) {
break; // Exit the loop if someCondition is true
}
} while (condition);
Break code : Switch Statement
int day = 3;
String dayName;
switch (day) {
case 1:
dayName = "Sunday";
break;
case 2:
dayName = "Monday";
break;
case 3:
dayName = "Tuesday";
break;
case 4:
dayName = "Wednesday";
break;
case 5:
dayName = "Thursday";
break;
case 6:
dayName = "Friday";
break;
case 7:
dayName = "Saturday";
break;
default:
dayName = "Invalid day";
break;
}
System.out.println(dayName);
Break code : For loop
for (int i = 0; i < 10; i++) {
if (i == 5) {
break; // Exit the loop when i is 5
}
System.out.println(i);
}
Continue keyword
Continue keyword is also the part of flow control statements in java. By using continue keyword in java we can skip the itterration for the current ongoing loop. For example I designed a loop to print 1 to 50 numbers. But I don’t want to print the number 5. Now how we can achieve this goal ? Obviously to achieve this we will use continue keyword by checking and condition that current index is qual to 5 or not. Let’s take the same example in the code below so you will understand this with ease.
Syntax
for (initialization; condition; update) {
// Code to execute in each iteration
if (someCondition) {
continue; // Skip the rest of the loop's body and continue with the next iteration
}
// Other code in the loop
}
Continue code
public class Main {
public static void main(String[] args) {
// Loop from i = 0 to i = 50 (inclusive)
for(int i = 0; i <= 50; i++){
// Check if i equals 5
if (i == 5){
continue; // Skip the rest of the loop body and continue with the next iteration
} else {
System.out.println(i); // Print the value of i if it is not equal to 5
}
}
}
}
Loops Statements
Loops are the important part when it comes to doing repetitive work. By using loops we can perform any kind of work again and again without writing the same code again and again. There are 4 types of loops exists in java first one is “for loop” second is “While loop “ third is “Do while loop” and fourth is “for each loop”. If you want to study loops in more detail then you can click on this link and study about loops in full detail with examples.
Conclusion
So I hope this article helped you guys to learn about control statements in java in detail. I tried my best to teach you guys about control statements and its use cases and types. Still if you have any question or issue then comment section is all yours. Please share your thoughts and suggestion. We would love to know and help you guys. If you have any suggestion you can connect with us on suggestions@thecseguide.com or can contact us. I will see you guys in next exciting article till then bye!!!