Table of Contents
Introduction
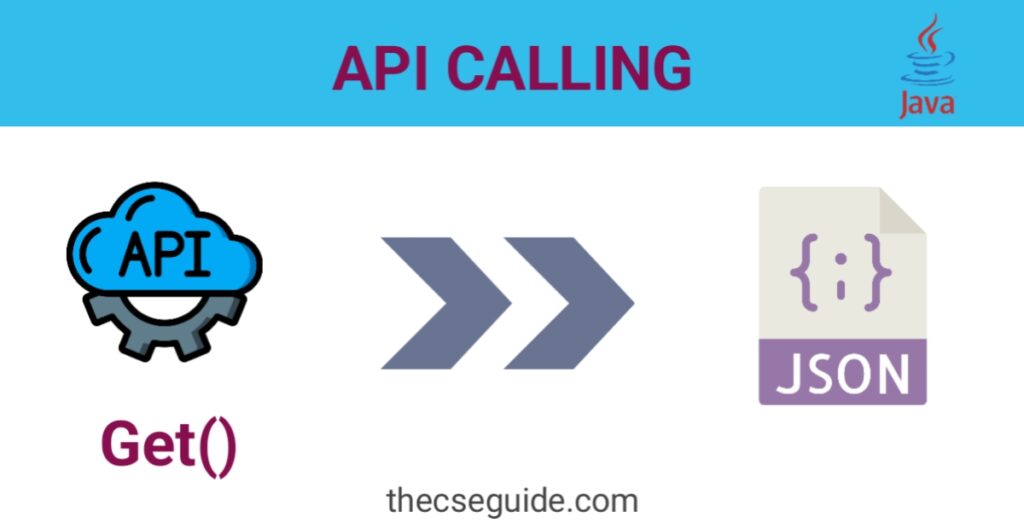
Hello Everyone, Today in this article we are going to learn how to call api in java with “GET” request. The Api I am using is publicly available. The api we are using in this article will give you url of a random dog image. You can you this reference GitHub link to choose the api of you choice on which you want to work. In this article we are not using any method which requires third party library to call api. So let’s get started and learn about how to call api in java.
Methods to call api
Using HttpClient
Note : Make sure you will have JAVA 11 or higher version installed to use HttpClient.
Algorithm
- First create an instance of HttpClient.
- Now Create HttpsRequest Builder and then pass desired url in the form of URI.
- Select the Desired method in this case we are using GET method.
- Now send request by using HttpsClient instance.
- Check if the response this ok by checking if the status code of api is 200 or not.
- If the response code is 200 then use the result. Else print an error message to the user.
Code
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
public class DogApiExample {
public static void main(String[] args) {
try {
// Create an HttpClient instance
HttpClient client = HttpClient.newHttpClient();
// Create a HttpRequest instance
HttpRequest request = HttpRequest.newBuilder()
.uri(new URI("https://dog.ceo/api/breeds/image/random"))
.GET()
.build();
// Send the request and get the response
HttpResponse<String> response = client.send(request, HttpResponse.BodyHandlers.ofString());
// Check if the response is successful
if (response.statusCode() == 200) {
System.out.println(response.body());
} else {
System.out.println("GET request failed. Response Code: " + response.statusCode());
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Using HttpURLConnection
Algorithm
- First define a URI.
- Convert URI to URL.
- Open the connection using HttpURLConnection and set method to GET.
- Check the response of the request. By using getResponseCode() method.
- If code equals to 200 then read the response. Else display error message to user.
- Create a BufferedReader tp read the input stream.
- Intialize StringBuilder.
- Read line by line using BufferedReader and append each line to StringBuilder.
- Close the BufferedReader.
- Convert StringBuilder content to string.
- Use the string content according to need.
Code
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URI;
import java.net.URL;
import java.util.logging.Level;
import java.util.logging.Logger;
public class DogApiExample {
private static final Logger logger = Logger.getLogger(DogApiExample.class.getName());
public static void main(String[] args) {
try {
// Define the URI
URI uri = new URI("https://dog.ceo/api/breeds/image/random");
logger.info("URI created: " + uri);
// Convert the URI to a URL
URL url = uri.toURL();
// Open a connection to the URL
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
// Get the response code
int responseCode = connection.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) { // success
BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String inputLine;
StringBuilder response = new StringBuilder();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
// Print the response
String responseBody = response.toString();
System.out.println(responseBody);
} else {
logger.warning("GET request failed. Response Code: " + responseCode);
}
connection.disconnect();
} catch (Exception e) {
logger.log(Level.SEVERE, "Exception occurred", e);
}
}
}
These are the two methods which do not require any third party library to call api. There are some other alternative libraries also available to perform api calling. For example JSOUP.
Output
After running the above code you will get output like this.
{"message":"https:\/\/images.dog.ceo\/breeds\/chippiparai-indian\/Indian-Chippiparai.jpg","status":"success"}
Conclusion
So I hope this article helped you guys to learn about how to call api in java in detail. I tried my best to teach you guys about api calling in java. Still if you have any question or issue then comment section is all yours. Please share your thoughts and suggestion. We would love to know and help you guys. If you have any suggestion you can connect with us on suggestions@thecseguide.com or can contact us. I will see you guys in next exciting article till then bye!!!