Table of Contents
Introduction: Kotlin coroutines
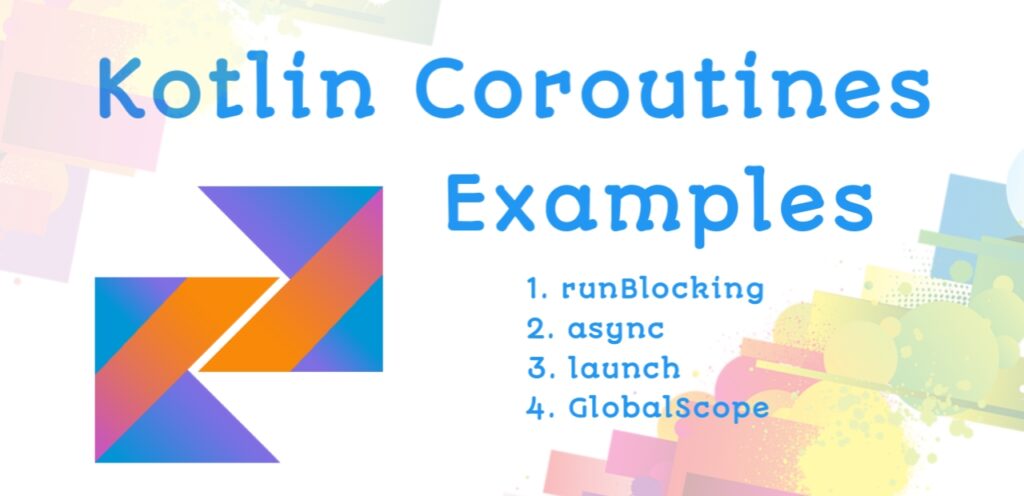
Hello, Everyone. Today, we will discuss a very important and fun topic, which is Kotlin coroutines. We will see everything about coroutines, and then we will take a look at coroutines and coroutines in Android examples. This article is going to be full of knowledge just read till the end. So let’s get started.
Q1. What is Kotlin coroutines?
Kotlin coroutines were introduced in version 1.3 and with the help of Kotlin coroutine, you can perform multiple tasks in the background without blocking the main thread. Kotlin coroutines are lightweight threads and easy to implement. it’s the feature with the help of that you can execute multiple tasks at the same time. Coroutines perform work in a defined scope which eliminates the possibility of memory leaks.
Q2. What’s the Use of Kotlin coroutines in Android?
In Android, every application has a main thread that is responsible for some of the actions that you can perform in an app, such as button clicks. This kind of task was handled by the Android main thread, but what if we want to download and file or load an image? Then, we cannot perform this kind of heavy task on the main thread because this will eventually throw an ANR (Application not responding) error, which makes the app entirely useless. So, to perform these heavy tasks without disturbing the main thread, we use Kotlin coroutines so that we can achieve concurrency without creating any issues in app performance.
Pre-requisite
Before starting the article, you need to add coroutines dependency in your IDE or Android studio. So that you can use the coroutine package In your code. To check the latest version of coroutines you can check out this article
Explanation: Kotlin coroutines example
Now, let’s move forward in this article and explore the power of Kotlin coroutines and understand more the Kotlin coroutines example. We will start from scratch, and then later on, we will raise the level. So, let’s begin.
1. GlobalScope.launch
GlobalScope.launch is a way to launch coroutine which is not bound to any specific lifecycle. This coroutine runs at the global scope of the application and will continue even if the calling activity is destroyed. Let’s take a look at the example to understand the practical usage better. If you have any UI-related work then using GlobalScope.launch is not recommended.
import kotlinx.coroutines.*
fun main() {
GlobalScope.launch {
delay(1000L) // Simulates a background operation
println("Hello from GlobalScope!")
}
println("Coroutine launched!")
Thread.sleep(2000L) // Keep the main thread alive to see coroutine output
}
// Output
Coroutine launched!
Hello from GlobalScope!
Now understand what happened in the above code First, we launched a coroutine to print “Hello from GlobalScope!” with a delay of 1000 milliseconds. Then, outside of that, we write a print statement to print “Coroutine launched!” and then use the “Thread.sleep” function to keep the main thread alive so we can see the coroutine output. Now, when we run the code, it will print the “Coroutine launched!
” text, and then after 1000 milliseconds, it will print “Hello from GlobalScope!”. But you know what if we still get the same result if I comment “Thread.sleep(2000L)”? Let’s try
// Output
Coroutine launched!
This will be the result, but why? I know you have this question, but if you remember, I told you before that GlobalScope.launch does not get affected by the application lifecycle, and the main thread doesn’t even know that it has to wait for the thread to complete its operation so it can end the program. The above code told the coroutines to wait for 1000 milliseconds to print the statement, and then by using thread, we keep the main thread alive for 2000 milliseconds so we can see the output of the coroutine.
But it’s quite boring, isn’t it? Adding wait time manually to keep the main thread alive so coroutines can finish their work, and it’s impossible to estimate the finish time when it comes to designing a real-world application. So, what can we do in this scenario? If you read the coroutines documentation, it states that GlobalScope.launch returns a “Job” By using this “Job”, we can inform the main thread to wait until the coroutines finish its work. Let’s take an example.
import kotlinx.coroutines.*
fun main() {
val job: Job = GlobalScope.launch {
delay(1000L) // Simulates a background operation
println("Hello from GlobalScope!")
}
// job.join() will inform the main thread to wait.
runBlocking { job.join() }
println("Coroutine launched!")
}
// Output
Coroutine launched!
Hello from GlobalScope!
Now, the output remains the same, but it’s a more dynamic approach to use coroutines as compared to manually adding a time. The “Job” also provides various functions, which you can explore by clicking on this.
2. runBlocking
The”runBlocking” is a blocking coroutines builder. It runs the coroutines in the current thread, which blocks the thread until all its code and child coroutines are finished executing. The “runBlocking” is typically used in main functions or testing environments to call suspending functions. For better understanding, let’s take an example.
import kotlinx.coroutines.*
fun main() {
println("Before runBlocking")
runBlocking {
launch {
delay(1000L) // Simulates a long-running operation
println("Inside runBlocking")
}
}
println("After runBlocking")
}
//output
Before runBlocking
Inside runBlocking
After runBlocking
While using “unlocking”, keep in mind that it blocks the main thread until it executes all its operations. I would suggest using “runBlocking” in the main function or for the testing of suspending functions.
3. launch
The launch is a coroutine builder used to start a new coroutine. The launch function is aware of the lifecycle. This means if an activity in Android got destroyed, then the coroutine created using the launch function will also be destroyed. The launch also returns a “Job” object, allowing us to control the coroutine in various manners. I think we should take an example to understand better. This coroutine builder is also known as fire and forget coroutine builder.
import kotlinx.coroutines.*
fun main() = runBlocking { // Creates a CoroutineScope tied to the main thread
launch {
println("Hello from launch!")
}
println("Coroutine launched!")
}
//Output
Coroutine launched!
Hello from launch!
Use launch when you want to do some work that can be done within the scope of the lifecycle. This will help you to prevent leaks and crashes.
4. async
The async coroutine builder is quite similar to the launch coroutine builder but with a little difference. Similar to the launch coroutine function, this async coroutine will inherit the coroutine scope from the immediate parent coroutine. But the difference between async and launch is that async returns a “Deferred” object, Which is the subclass of the “Job” class. In comparison, the launch returns a “Job” object. Now, if we want any return value from our coroutine, then we can use an async coroutine. To use this async at the global or application level, use “GlobalScope.async” Let’s better understand async and GlobalScope.async with an example.
import kotlinx.coroutines.*
// async example
fun main() = runBlocking {
println("Inside main")
val jobDeferred : Deferred<String> = async {
"I came from inside of async coroutine"
}
val value = jobDeferred.await()
println(value)
println("Exit main")
}
//Output
Inside main
I came from inside of async coroutine
Exit main
import kotlinx.coroutines.*
// GlobalScope.async
fun main() {
println("Inside main")
val jobDeferred : Deferred<String> = GlobalScope.async {
"I came from inside of GlobalScope.async coroutine"
}
runBlocking {
val value = jobDeferred.await()
println(value)
}
println("Exit main")
}
// output
Inside main
I came from inside of GlobalScope.async coroutine
Exit main
Conclusion
So, I hope this article helped you guys to learn about the Kotlin coroutines. I tried my best to teach you guys about it. But Still, if you have any questions or issues, then the comment section is all yours. Please share your thoughts and suggestions. We would love to know and help you guys. If you have any suggestions, you can connect with us at suggestions@thecseguide.com or contact us. I will see you guys in the next exciting article till then, bye!!!