Table of Contents
Introduction
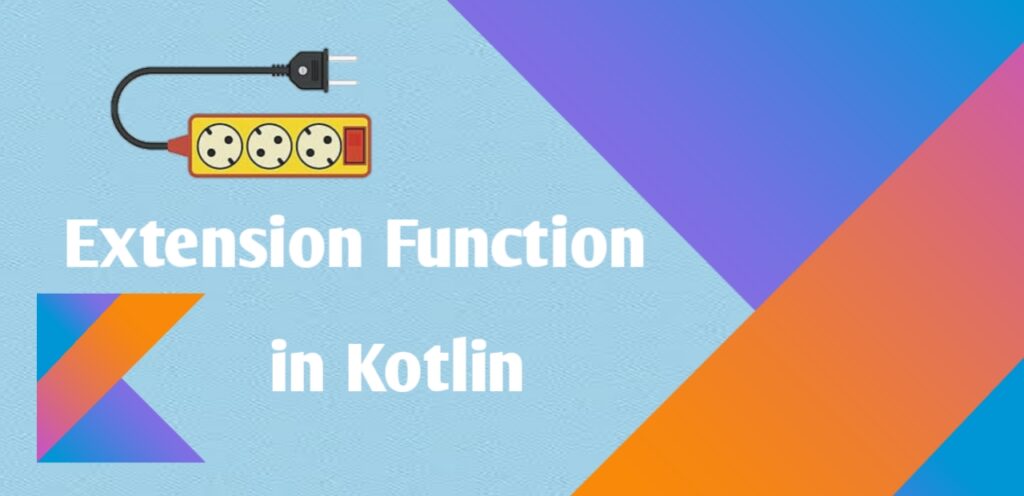
Hello Everyone, Today in this article we will discuss on a very exciting topic called the Kotlin extension function. We will discuss its needs and use cases in depth. I will explain everything in detail so be ready to explore Kotlin extension function. so let’s get started.
What is Kotlin extension function
Kotlin extension functions are used to introduce a new function in pre-defined and custom classes. Kotlin extension function is a member function of a class that is created outside the class. The extension function is defined with a receiver type and a receiver object. These functions provide an efficient way to extend the functionality of a class inside which they are defined.
Examples
Extension function using predefined class
fun main(){
val name = "Akash"
val mam = "Madam"
println(name.isPalindrome())
println(mam.isPalindrome())
}
private fun String.isPalindrome() : Boolean{
return this.lowercase() == this.lowercase().reversed();
}
In the above example, we created an extension function from a pre-defined class known as String. The extension function we created is called ‘palindrome’. This function checks whether the given string is a palindrome. We take two strings: the first is “Akash,” and the second is “Madam.” Now, have you noticed something? If not, then take a look at the ‘palindrome’ function; this function uses the String class to determine its behaviour. This means that this function now knows it must deal with the String datatype. The mind-blowing thing about this is that the String class doesn’t have the ‘palindrome’ method, and we created this method without making any changes to the pre-defined String class.
Extension function using a custom class
The code example 1.1 below contains a class named Square, and the Square class has a function called Area. Now, what if we want to create a function called to calculate the perimeter of the square without defining the method in the Square class? Now, to solve this problem, we will use the Kotlin extension function.
fun main(){
val square = Square()
square.Area(2)
}
class Square {
fun Area(value : Int): Int{
return value * value
}
}
Example 1.1: Custom class without extension function
fun main(){
val square = Square()
square.Area(2)
square.isPerimeter(2)
}
class Square {
fun Area(value : Int): Int{
return value * value
}
}
fun Square.isPerimeter(value : Int): Int{
return 4 * value
}
Example 1.2: Custom class with extension function
The code example 1.2 contains the same code from example 1.1, But this example contains the Kotlin extension function. If you take a close look the “isPerimeter” function is not defined inside the Square class, but still, we can use the “isPerimeter” function from the object of the square. We can achieve this only with the help of the Kotlin function.
Swap elements in the array list
Now, we will use the Kotlin extension function to swap element positions in an ArrayList. In this example, we will create an extension of the ArrayList class. Take a look at the below code so you can understand the concept more clearly.
// ArrayList<String>.swap is used to create extension function
fun ArrayList<String>.swap(i: Int, j: Int) {
val temp = this[i]
this[i] = this[j]
this[j] = temp
}
fun main() {
//Create an array list with data
val names = arrayListOf("Rohit", "Sonam", "Akshay")
// call the swap extension function
names.swap(0, 1)
// Output will be [Sonam, Rohit, Akshay]
println(names)
}
Conclusion
So, I hope this article helped you guys to learn about the Kotlin extension function in detail. I tried my best to teach you guys about The Kotlin extension function and its use cases. Still, if you have any questions or issues, then the comment section is all yours. Please share your thoughts and suggestions. We would love to know and help you guys. If you have any suggestions, you can connect with us at suggestions@thecseguide.com or contact us. I will see you guys in the next exciting article till then, bye!!!