Table of Contents
Introduction: Kotlin vs Java
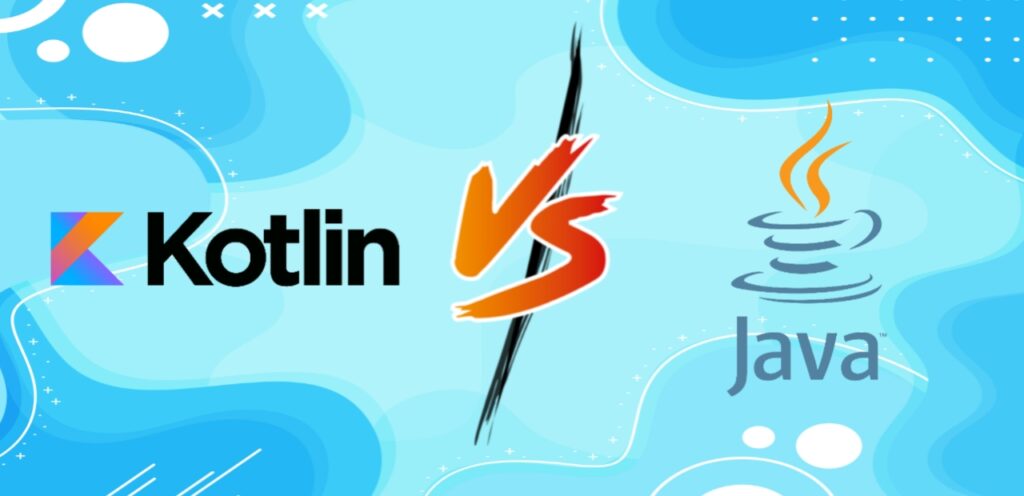
Hello, Everyone. Today, we will see the syntax difference between Kotlin vs Java. I designed this article while keeping this in mind so that this comparison between Kotlin vs Java for beginners will be easy to understand. I think this article will be helpful for you if you are trying to migrate from Java to Kotlin. I will explain to you everything is easy, So your journey from Java to Kotlin has become smoother. So, let’s get started
What is Kotlin
Kotlin is a modern, statically typed programming language developed by JetBrains and officially released in 2011. It is based on Java Virtual Machine (JVM) and is fully interoperable with Java. Kotlin is designed to improve developer productivity by providing concise, expressive, and safe code. It supports functional programming features and is commonly used for Android app development. Kotlin also launched Kotlin Multi-platform in 2017 which enables developers to develop apps across different devices for example IOS, Android, Web etc.
Kotlin vs Java Differences
Difference 1: Variable Declaration
In Java, to define a variable name, we first write the datatype, then the variable’s name, and then assign a value to it. To understand better take a look at below Java code.
// Java Variable Declaration
public void main(){
String Name = "Akash";
int Age = 21;
Double Salary = 33234.5;
}
Example: Variable declaration in Java
In Kotlin the variable declaration is quite interesting. Let’s break down it one by one. Did you know? In Kotlin, you can Directly assign a value to a variable without defining the datatype of that variable. Of course, you can define the datatype of the variable in the beginning as well. Let’s understand everything with practical code.
fun main(){
// Kotlin will automatically assume that Akash is an string and define String Datatype to it, Same goes for Salary, Age
var Name = "Akash"
var Salary = 33234.5
var Age = 21
}
Example: Variable declaration without defining datatype
fun main(){
// Kotlin Variable Declaration With datatype
var Name: String = "Akash"
var Salary: Double = 33234.5
var Age = 21
}
Example: Variable declaration with defining datatype
Difference 2: Function Declaration
The syntax to define a function is a bit fun and easy as compared to Java. Let’s first take a look at Java code for declaring a function. Then we will move to Kotlin code.
// Java code
// Method with string return type
public String First() {
return "Hello Its function";
}
// Method Without return type and parameter
public void Second(){
// Write code here
}
// Method with parameter
public void Third(String value){
// Use value according to need
}
Example: Function Declaration with or without return type and parameter
In Java, while declaring a function, first, we write the return type and then provide the function name with an open and closed bracket “()” inside this bracket, we can define the parameters of the function, then add an open and closed curly bracket “{}”, and inside that, we write the code.
Now, Let’s talk about the Kotlin way of declaring a function. In Kotlin, we use the “fun” keyword to define a function, and after this, we write the function name with open and closed brackets “()” In between these brackets, we can write a provide function parameter, and then we use “:” this sign and then define a return datatype else we write open and close curly bracket “{}”. Then, inside this, we write code. Let’s take a look at this example in a practical way.
// Kotlin code
// Method with string return type
fun First() : String {
return "Hello Its function"
}
// Method without return type
fun Second() {
// Write code here
}
// Method with parameter
fun Third(name : String) {
// Write code here
}
// Method with default parameter value
// This option is not available in Java
fun Fourth(name : String = "Rahul") {
}
Example: Function Declaration with or without return type and parameter
Difference 3: final vs val
If you have ever worked with Java, then you also used the” final” keyword in your Java codebase. In Kotlin, the “val” does the same work as the “final” keyword does in Java. But somehow, if you forgot the usage of the “final” keyword, then I will make you remind that if we want to define the variable value only once, we don’t want this value to be changed anywhere in the code. Then, we use the “final” keyword in Java and val in Kotlin to achieve this. Now, take a look at Java and Kotlin code to understand the concept practically.
// Java Code
public void main(){
/*
This code will throw an error that Name cannot be reassigned.
If you want to see the error Remove the comment to see
final String Name = "Akash";
Name = "Rohit";
*/
/*
But If you take a look the value of age can be easily changed later on from 21 to 22.
Because it's not defined using final.
int Age = 21
Age = 22
*/
}
Example: “final” keyword demonstration in Java”
// Kotlin Code
fun main(){
/*
This code will throw an error that Name cannot be reassigned Remove the comment to see
val Name: String = "Akash"
Name = "Rohit" */
/*
But If you take a look the value of age can be easily changed later on from 21 to 22.
Because it's defined using var.
var Age = 21
Age = 22 */
}
Example: “val” keyword demonstration in Kotlin”
Difference 4: Null safety
Java does not provide any null safety-like feature. In Java, we have to check if the value is null or not manually, and it makes it hard for a coder to manually check every time for a null value where the developer is expecting that the code can get a null value as a result. But Kotlin gave us a Null safety feature with “?” this sign. Let’s see this thing practically through code.
// Java code
String name = null; // Can throw NullPointerException
if (name != null) {
System.out.println(name.length());
}
Example: Java way of handling Null value
// Kotlin Code
// ? this sign inform the variable that incoming value might be null
val name: String? = null
println(name?.length) // Safe call operator
Example: Kotlin way of handling Null value
Difference 5: switch vs when
The fifth difference between Kotlin vs Java syntax is in Java, we use switch and case keywords to choose the option and code accordingly. For example, if I want to check what’s the current day and want to print the current day on the console. Then, in this scenario, I can use a switch case in Java. Because we have multiple choices and choose one according to the goal. Now, what if I want to perform the same action in Kotlin? Then Kotlin gives us “when” keyword. Let’s see the code to understand the syntax difference more clearly.
// Java code
public void main(){
String week = "Sunday";
switch (week){
case "Sunday":
System.out.println("Sunday");
break;
case "Monday":
System.out.println("Monday");
break;
case "Tuesday":
System.out.println("Tuesday");
break;
}
}
Example: Switch case in Java
// Kotlin code
fun main (){
var day = "Sunday"
when (day){
"Sunday" -> println("Happy $day")
"Monday" -> println("Happy $day")
"Tuesday" -> println("Happy $day")
"Thursday" -> println("Happy $day")
}
}
Example: “when” keyword usage in Kotlin
Difference 6: For Loop
In Kotlin vs Java syntax, the working principle of for loop is the same the only difference they have is in syntax. Both Kotlin and Java use the “for” keyword to initialise a loop. The for loop is much more concise and simpler in Kotlin as compared to the Java version. If you want to explore For Loop in full depth, then refer to the For Loop Article. Anyway, let’s take a look at the Java version of the for loop and loop in Kotlin then, we will see the for loop version of Kotlin.
// Java code
public void main(){
// print 1 to 10 using for loop
for (int i = 0; i <= 10; i++){
System.out.println(i);
}
}
Example: For loop in Java
//Kotlin code
fun main(){
// print 1 to 10 using for loop
for (a in 1..10){
println(a)
}
}
Example: For loop in Kotlin
Difference 7: Data class
How do you feel if I say you can create the Data class in just one line of code in Kotlin? Shocked right. But this is true. You can create a getter and setter for a data class with just one line of code. Whereas in Java you have to write the whole code for data class. For those who don’t know what a data class is in programming for them the simple definition is that “A Data class is used to set and get data. So that we can use it in our app.” Now first we will take a look at the Java version and then we take a look at the Kotlin version as well.
// Java code
public class User {
private String name;
public User(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
Example: Data (Pojo) class in Java
// Kotlin code
data class User(val name: String)
Example: Data (Pojo) class in Kotlin
Difference 8: Collections
Kotlin provides built-in functions and a more concise syntax for collections, whereas Java relies on utility methods from libraries like collections. Let’s take a look at the Java and Kotlin code to understand the concept more clearly.
// Java code
import java.util.Arrays;
import java.util.List;
public void main(){
List<String> names = Arrays.asList("Akash", "Bob Marley");
}
Example: Array initialisation in Java
// Kotlin code
fun main(){
val names = listOf("Akash", "Bob Marley")
val age = arrayOf(21,32)
}
Example: List and Array initialisation in Kotlin
Difference 9: Inheritance
Similar to Java, Kotlin also has the concept of Inheritance, where code can inherit the properties of one class to another. But in Java, we use the extend keyword to inherit the class, and in Kotlin, we use “:” this sign. Let’s demonstrate How we can inherit the parent class For example, a Person in child classes named one is an Employee, and another is a Student.
// Kotlin code
open class Person(){
fun callPerson(message: String){
println(message)
}
}
Example: Parent class called Person
// Java code
public class Student extends Person {
public void main(){
callPerson("Hey I am main method of Student calling Person Class");
}
}
Example: Child class named Student inherited from the Person class
// Kotlin code
class Employee : Person(){
fun main(){
callPerson("Hey I am main method of Employee calling Person Class")
}
}
Example: Child class named Employee inherited from the Person class
Difference 10: Extension function
Kotlin introduced the concept of the extension function, which enables the developer to create a function from a pre-defined and custom class without making changes in the original class. Java does not have any concept like an extension function. Now, let’s take a look at how the code for the extension function looks. If you want to study extension functions in depth, then you can read this article.
// Kotlin code
fun main(){
val name = "Akash"
val mam = "Madam"
println(name.isPalindrome())
println(mam.isPalindrome())
}
private fun String.isPalindrome() : Boolean{
return this.lowercase() == this.lowercase().reversed();
}
Example: Extension Function in Kotlin
Conclusion
So, I hope this article helped you guys to learn about the Kotlin extension function in detail. I tried my best to teach you guys about The Syntax difference between Kotlin vs Java. Still, if you have any questions or issues, then the comment section is all yours. Please share your thoughts and suggestions. We would love to know and help you guys. If you have any suggestions, you can connect with us at suggestions@thecseguide.com or contact us. I will see you guys in the next exciting article till then, bye!!!